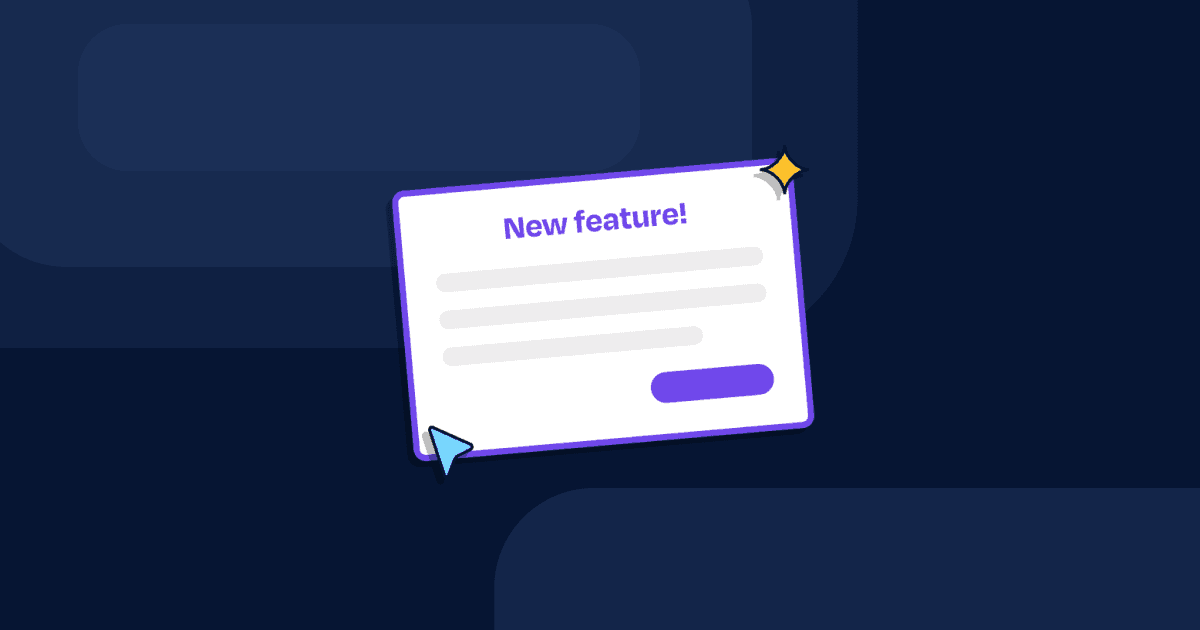
Announcing in-app announcements
We’ve added support for making in-app announcements without code changes! First, use Dopt to develop an in-app modal, card, fly-out (or anything) and then launch announcements through it without a dev.
This enables you to announce new products or features, communicate changes, notify users, and promote events in your product.
To deploy self-serve in-app announcements, you use the new Restart all users version transition combined with the latest
version tag in your code.
Read the guide on launching in-app announcements in our docs →
New in-app getting started tutorial
Our new getting started tutorial helps you learn the basics of Dopt by creating an example app that uses our new pre-built components and rich text content. The example flow is now created on demand rather than automatically, helping to keep your workspace tidy.
You can access the in-app getting started tutorial via the main nav.
See a live version of the example app you create in the tutorial →
Easier user and group identification in React
We shipped @dopt/react-users
, a React-specific utility for identifying users and groups to Dopt. The goal was to make the identification of users and initialization of our React SDK dead simple. See a typical usage in our example code here.
The packages provides a DoptUsersProvider
that you initialize with your User API key. Here’s an example:
<DoptUsersProvider apiKey={DOPT_USERS_API_KEY}> <App /></DoptUsersProvider>
Inside the <App />
from above, you can now use the package’s hooks, identifyUser
and identifyGroup
. Here’s an example of identifyUser
:
export function App() { // Identify an example user to Dopt the first time the App loads. const userId = useIdentifyUser({ identifier: nanoid(), properties: { company: 'Dopt', role: 'admin', inTrial: true, }, });
return ( <DoptProvider apiKey={DOPT_BLOCKS_API_KEY} userId={userId} flows={{ 'custom-card-component': 1 }} > <Home /> </DoptProvider> );}
The hook will correctly return undefined for the userId
until the request has been made successfully, which allows the <DoptProvider />
to initialize correctly!
We've been using this wrapper package all over the place in our own examples and internal tests and found it super useful, so excited to ship it for all of you.
In-app feedback
You can now give us feedback from in the product! You can find the feedback form in the main nav. We look forward to hearing from you 😄.
Other improvements
- We’ve defaulted path names to
complete
making it easier to create paths from custom blocks. You can still rename the path to whatever you want! - We now only allow one menu and popover to be opened at time, simplifying the UI.
- We fixed a bug where we weren’t redirecting users to the page they were trying to access if they happened to be logged out. You’ll now be redirected to the right page.